30. April 2024
How to Develop Kubernetes Operators from the Ground Up
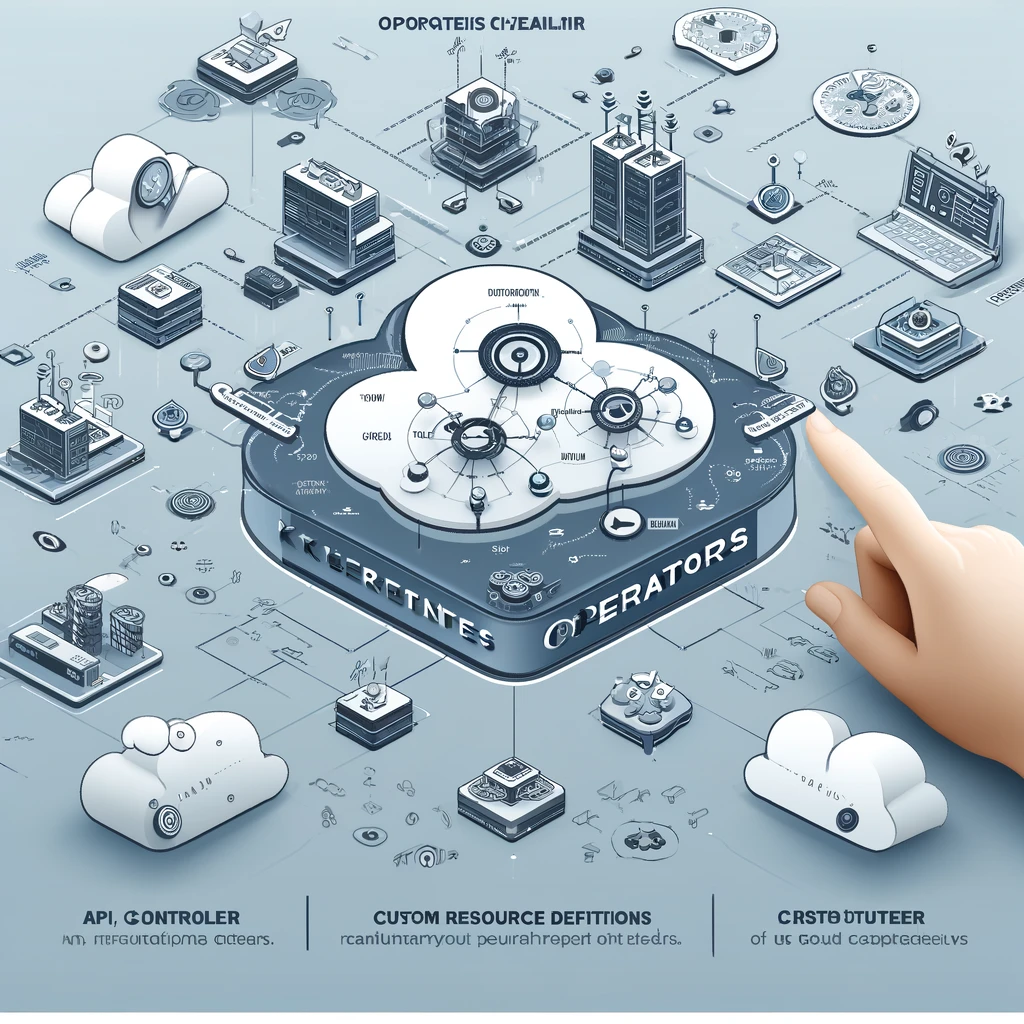
Introduction
Kubernetes operators are software extensions that make use of the Kubernetes API to manage applications and their components. They help automate the lifecycle of complex software, making Kubernetes clusters easier to manage with less manual intervention. In this post, we’ll guide you through the process of developing a Kubernetes operator from scratch.
What is a Kubernetes Operator?
A Kubernetes operator is essentially a method of packaging, deploying, and managing a Kubernetes application. A Kubernetes application is both deployed on Kubernetes and managed using the Kubernetes APIs and kubectl tooling. Operators simplify complex software operations, such as upgrades, backups, and scaling.
Step 1: Understand the Basics
Before diving into the development, it’s crucial to understand the core concepts of Kubernetes like Pods, Services, and StatefulSets. Familiarize yourself with the Kubernetes documentation.
Step 2: Setting Up Your Development Environment
- Install Tools: Ensure you have
kubectl
, and the Kubernetes cluster is accessible. Also, install the Operator SDK, which provides tools to help you write, build, and run Operators. - Create a Project: Use the Operator SDK to create a new project. For example:
1operator-sdk init --domain=example.com --repo=github.com/example/myoperator
Step 3: Create a Custom Resource Definition (CRD)
Operators work by extending Kubernetes APIs with Custom Resource Definitions (CRDs). Define your CRD to specify the desired and actual state of your application components.
1apiVersion: apiextensions.k8s.io/v1
2kind: CustomResourceDefinition
3metadata:
4 name: myresources.example.com
5spec:
6 group: example.com
7 versions:
8 - name: v1
9 served: true
10 storage: true
11 schema:
12 openAPIV3Schema:
13 type: object
14 properties:
15 spec:
16 type: object
17 properties:
18 size:
19 type: integer
20 name:
21 type: string
Step 4: Implement Your Operator Logic
Write the operator’s logic to handle the application lifecycle based on your CRD. Implementing operators often involves watching for changes to your custom resources and managing resources accordingly.
1import (
2 "context"
3 "github.com/operator-framework/operator-sdk/pkg/log/zap"
4 "sigs.k8s.io/controller-runtime/pkg/client"
5 "sigs.k8s.io/controller-runtime/pkg/controller/controllerutil"
6 "sigs.k8s.io/controller-runtime/pkg/reconcile"
7)
8
9func (r *Reconciler) Reconcile(ctx context.Context, req reconcile.Request) (reconcile.Result, error) {
10 // Operator logic here
11}
Step 5: Build and Deploy Your Operator
Once you’ve implemented the logic, build and deploy your operator. Ensure it’s tested thoroughly.
1operator-sdk build my-operator-image:tag
2kubectl apply -f deploy/operator.yaml
Conclusion
Developing Kubernetes Operators can greatly simplify the management of applications on Kubernetes by automating routine tasks. Follow these steps to start creating your own operators and improve the scalability and reliability of your systems.
For more detailed guidance and advanced topics, refer to the Operator SDK User Guide.
In the next post, I’ll be guiding how to create your first Kubernetes Operator.